Implementing the iFrame Solution [V.1 Deprecated]
The iframe solution offers a simple option to partners who are only selling Viator experiences and who don’t want the overhead of dealing with their customer payment details.
Note: this is v.1 iFrame. For the latest version, please see here.
iFrame example
Here is an example of what the iFrame generally looks like.
The iFrame can be styled, as described in our styling guide.
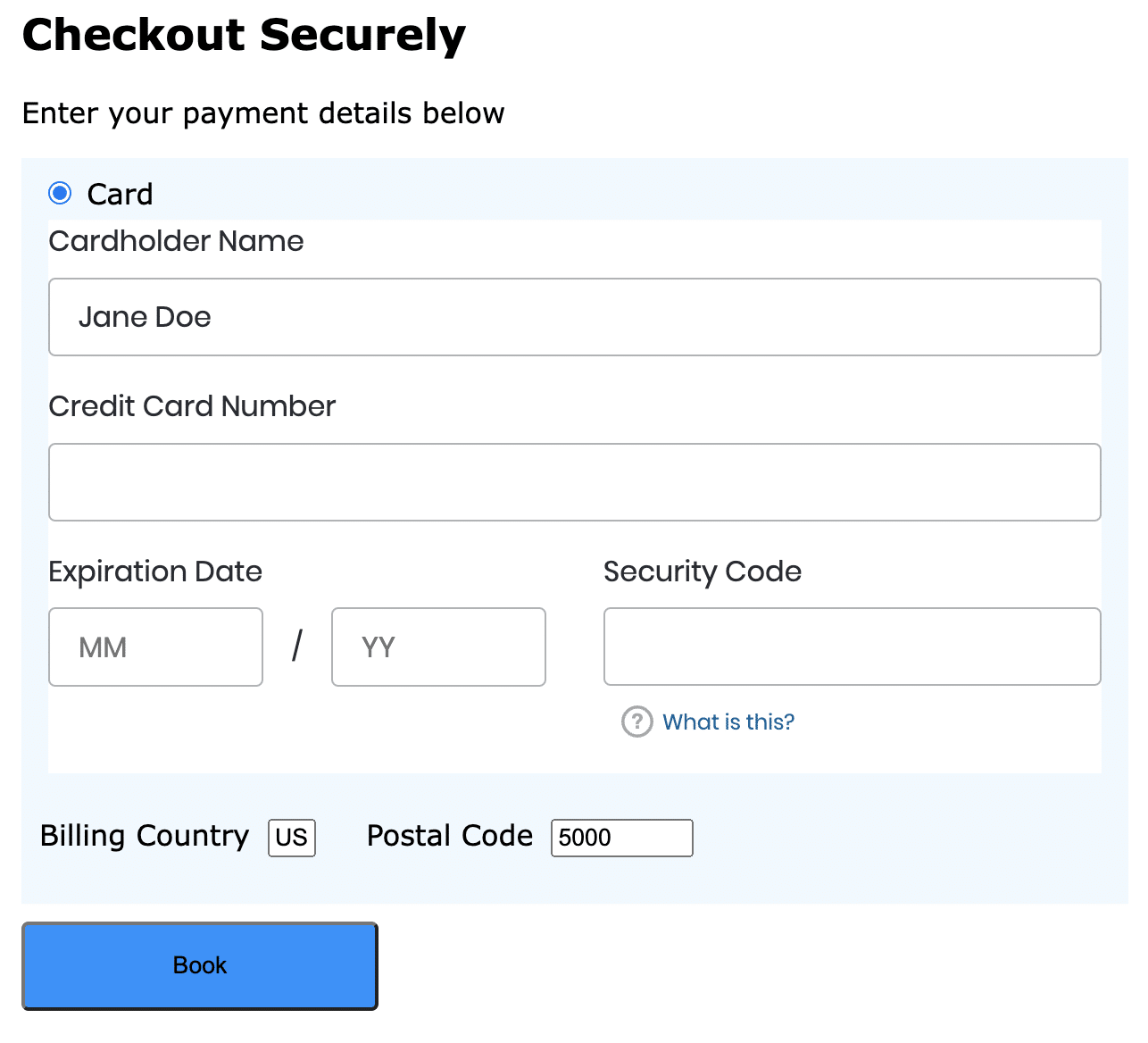
Prerequisites
Content Security Policy
Partners who implement a Content Security Policy on their sites must permit resources for script-src and frame-src directives on the page where the iframe will be included.
The script-src directive specifies valid sources for Javascript, allowing the Viator library to initialize the iframe and control the interactions between the iframe.
The frame-src directive specifies valid sources for the iframe element allowing communication with the Viator servers.
Directive | Sandbox Host | Production Host |
frame-src | https://api.staging.tapayments.com | https://api.tapayments.com |
script-src | https://checkout-assets.payments-dev.tamg.cloud | https://checkout-assets.payments.tamg.cloud |
Example Content Security Policy for Production:
Content-Security-Policy: script-src
https://checkout-assets.payments.tamg.cloud; frame-src
https://api.tapayments.com
Browser Support
The iframe solution supports the latest versions of Edge, Firefox, Chrome and Safari.
iFrame Implementation
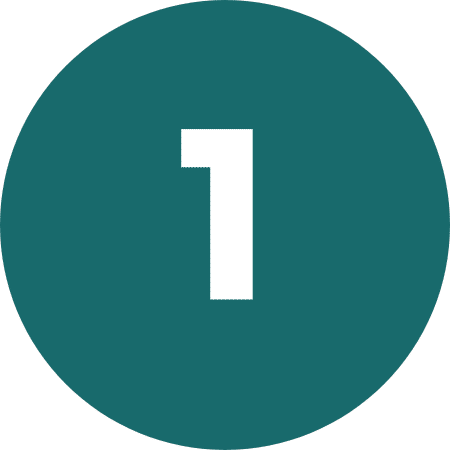
Check Availability and Pricing
Endpoint: /availability/check
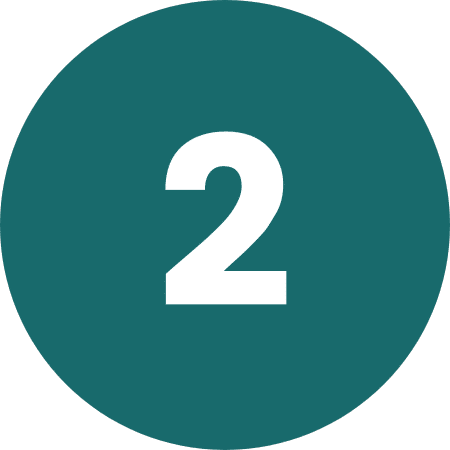
Request a Booking Hold:
Endpoint: /bookings/cart/hold
Note: The payment session token returned from this call is valid for a period of 1 hour after which it will expire. This token is required to be valid for calling the Confirm the Booking endpoint.
iFrame flow specific request values:
Property | Value |
paymentDataSubmissionMode | VIATOR_FORM |
hostingUrl | Provide the full URL to your checkout page where the iframe will be hosted. |
iFrame flow specific response values:
Property | Value |
paymentSessionToken | The payment session token is used to initialize the iframe. |
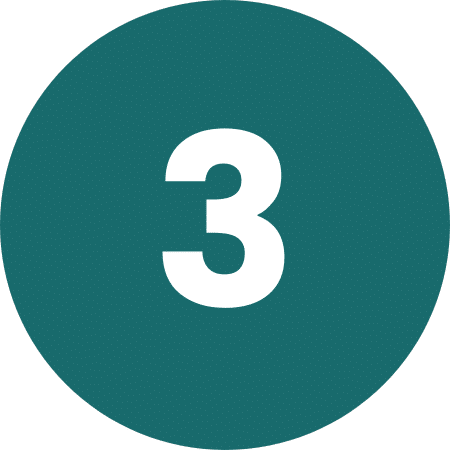
Payment
iFrame flow success response values:
Property | Value |
paymentToken | The payment token for the payment details. Required in the confirm booking endpoint call. |
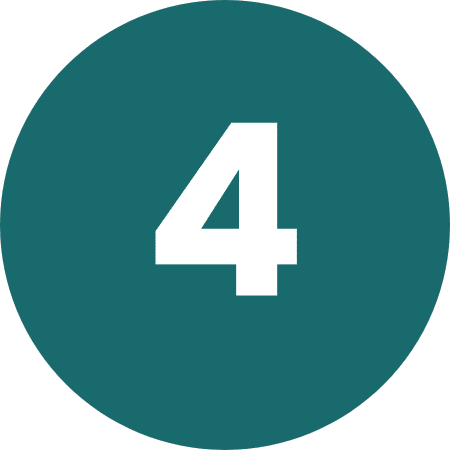
Confirm the Booking
Endpoint: /bookings/cart/book
iFrame flow specific request values:
Property | Value |
paymentToken | The paymentToken returned in the object passed to the success callback method from the payment submitForm call. |
Viator Javascript Library
The Viator Javascript library must be imported into your payment details page using the following URL:
https://checkout-assets.payments.tamg.cloud/stable/v1/payment.js
Step-by-Step Guide
These steps will guide you through implementing the Viator iframe payments solution within your checkout page. This guide covers the main aspects that are required and should give you enough of an idea to adjust, as needed, to support your use case.
Step 1
On the page where payment details will be collected, in the <head> section of the page, include the Viator UI Javascript library. This library is required to initialize the iframe.
Note: Use the correct URL per the table above for sandbox and production environments.
<script src=“https://checkout-assets.payments.tamg.cloud/stable/v1/payment.js“></script>
Step 2
Include an element where you want the payment details iframe to be injected. The element’s id will reference this element in the initialization Javascript.
<div id=“payment-iframe-holder”>
<!– Secure iframe is injected here –>
</div>
Step 3
Provide initialization logic which will be called on page load with the paymentSessionToken that was returned from the /bookings/cart/hold API endpoint.
The element id from Step 2 should be used as the value for the cardElementContainer.
The payment variable should be in a scope that can be called during form submission.
See the Styling section for full styling options.
var payment;
function initPaymentIframe(paymentSessionToken) {
payment = new Payment(paymentSessionToken);
payment.renderCard({
cardElementContainer: ‘payment-iframe-holder’,
onFormUpdate: onFormUpdate,
styling: {
variables: {
colorBackground: ‘#FFF’,
colorPrimaryText: ‘#000‘
}
}
});
}
Step 4
On page load, call the method to initialize the iframe passing in the payment session token returned from the /bookings/cart/hold API endpoint.
<body onload=“initPaymentIframe(‘$paymentSessionToken’)”>
Step 5
Provide the form submission logic for your payments page, which should be called to process the payment details before you handle the processing of the form.
The onSubmit method should be called when the form is submitted, and call the submitForm method of the payment variable defined in Step 3. The argument to submitForm is an object with an address field containing fields for the payer’s country code and postcode/zip code.
The onSubmitSuccess and onSubmitError methods will be called on success and error, respectively, from submitting the payment details to the payment gateway. These methods must be implemented appropriately for dealing with successful or error responses.
The payer’s country code and postcode are not included in the iframe collection form and must be collected by the partner to submit the payment details.
Note: The country code is the alpha-2 code for the customer country.
The onSubmitSuccess callback method will be passed an object in the following format:
{
result: “SUCCESS”,
paymentToken: <Token>,
cardData: {
cardType: “Visa”
}
}
The paymentToken is required for the Confirm the Booking endpoint.
function onSubmit(e) {
// omitted … read country and zip fields on page
const bodyData = {
address: {
country: country,
postalCode: zip
}
};
payment.submitForm(bodyData)
.then((res) => onSubmitSuccess(res))
.catch((err) => onSubmitError(err));
}
function onSubmitSuccess(message) {
// Handle success here
}
function onSubmitError(error) {
// Handle errors here
}
Step 6
When the form that contains the payment details is submitted, ensure that the form submission logic is called to process the payment details.
<button id=“submitBtn” type=“button” onclick=“onSubmit()”>Book</button>
Styling the iFrame
The styling of some elements within the iframe is supported. The full styling options available are listed below:
styling: {
colorTheme: ‘LIGHT|DARK’, // Defaults to LIGHT
variables: {
fontSize: ‘0.9rem’,
colorInputBackground: ‘#eee’,
colorBackground: ‘#eee’,
colorPrimaryText: ‘#212121’
}}
All styling settings are optional, with any combination of them supported. Colors can be a standard CCS name or HEX value. Font sizes must be in rem.
Complete Example
checkout.html
<html>
<head>
…
<script src=“https://checkout-assets.payments.tamg.cloud/stable/v1/payment.js“></script>
<!– checkout.js
<script src=“/checkout.js” type=“application/javascript”></script>
</head>
<body onload=”initPaymentIframe(‘$paymentSessionToken’)”>
…
<div>
<label for=“billing-country”>Billing Country</label>
<input type=”text” name=“billing-country” size=“2” width=“4” id=“billing-country” />
</div>
<div>
<label for=“zip”>Postal Code</label>
<input type=“text” name=“zip” value=“5000” size=“10” id=“zip” />
</div>
<div>Enter your payment details below</div>
<div id=“payment-iframe-holder”>
<!– Secure iframe is injected here –>
</div>
<button id=“submitBtn” type=“button” onclick=“onSubmit()”>Book</button>
…
</body>
</html>
checkout.js
var payment;
function initPaymentIframe(paymentSessionToken) {
payment = new Payment(paymentSessionToken);
payment.renderCard({
cardElementContainer: ‘payment-iframe-holder’,
onFormUpdate: onFormUpdate,
styling: {
variables: {
colorBackground: ‘#FFF’,
colorPrimaryText: ‘#000’
}
}
});
}
function onSubmit(e) {
// omitted … read country and zip fields on page
const bodyData = {
address: {
country: country,
postalCode: zip
}
};
payment.submitForm(bodyData)
.then((res) => onSubmitSuccess(res))
.catch((err) => onSubmitError(err));
}
function onSubmitSuccess(message) {
// Handle success here
}
function onSubmitError(error) {
// Handle errors here
}